In a system where multiple types of energy source exist, running all components at all available times could be a waste by considering multiple different factors, including the device depreciation, normal energy in maintaining the entire system running. This waste is a significant factor when lower wind for wind producer happen, or lower light for light producer happen, or other stuff.
This is a javafx based energy running simulation simulator with choco-solver to find optimal threshold, which will be used to determine the time to turn on the device and time to turn off. It contains methods to analyze history data to predict a threshold to make the entire system running as efficient as it could. After proper data is provided, system will run based on a threshold setting from user or automatically analyzed based on history data so that no energy will be wasted. This app is done solely by me.
Ways of analyzing history data
If Using the first 30 input data to optimize and get the best possible threshold.
is checked, the first 30 data will be used to generate the optimized threshold.
By comparing methods in passage Optimal Energy Production designed by me and the usage case, the following one is chosen at last:
Define variables
J : type of energy, {wind, water, }
N : value index, 1, 2, .
C = : the normal energy consumption per unit time for each producer (Need to consider as a combination of multiple equipments, like: if we have two wind machine(user is able to define this in control), each machine will consume 10 W. Then =10*2=20)
U = : Total user consumption
W = , the expected energy(total in above table) produced in each value index, either pre-calculated or calculate in model.
S = {0,1}, to indicate I should turn on wind on this statistic data or not
B : The battery charging limitation.
D , indicate the day count each value index, either pre-calculated or calculate in model.
Optimization Model
\begin{equation}\begin{split}&\text{Minimize } & \sum_{i\in N}\sum_{j\in J}D_{ij}S_{ij}\\& \text{Subject to}\ & 0 \le S_{ij} \le 1 \ \forall i\in N j\in J\\& & \sum_{i\in N}\sum_{j\in J}W_{ij}S_{ij}-\sum_{i\in N}\sum_{j\in J}C_{j}S_{ij}-U\ge B\end{split}\end{equation}Use MIP to solve it
Code to solve it
By comparing with multiple different libraries to solve it, choco-solver is used on java platform at last, which is able to solve problems more than optimization and use machine learning in some cases. It is also able to solve other machine learning problems and provide a good way to customize based on different personal usage cases.
This code including reading data from stream, which better fits the real situation. Such that streaming data would be easily handled in the real situation. Details core code has multiple methods and it is visible at the Github repo.
Running
A easy way is to download the execution file from the publication page at Release, be caution that this is generated using Java, so only use it for study purpose.
Compile and run from source
The source code is located at https://github.com/ShaokangJiang/energy-javafx, it is tested to be working with JFoenix, choco-solver, JavaFX8, Java JDK.
NOT RECOMMEND as too many arguments, dependencies and it is easy to be wrong
Data format:
Simulation data
Header should be the same, User_Usage
is not required but recommend. Each field represent the value sensor received.
1 | Time,Wind_Speed,Light_H,Wave_Hight,Wave_Period,Current_Speed,User_Usage |
Download a sample from here.
Optimization data
Header should be the same, idx
is not required. Each category has a value component recoding the amount of power produced in unit time. Each count means the possibility of happening. Because we need to use a KWH = b KW * s/3600 to calculate energy produced. The count field has to be integer. We could use the possibility * a factor to make it become integer. And the sum of each count should be the same.
1 | idx,wind_value,wind_count,light_value,light_count,wave_value,wave_count,current_value,current_count |
Download a sample from here.
Generate Fake Simulation and optimization data
Fake simulation data
Use java code below to generate fake simulation data(to be used in run page).
1 | static Random r = new Random(); |
Fake optimization data
To make the input source be easy to manage, represent and maintain. We could save them in the same file, if no enough data for a cell, leave it empty would be fine and recommended.
idx is not required but recommended
This generation code might be good enough as this is not the real case
header: idx,wind_value,wind_count,light_value,light_count,wave_value,wave_count,current_value,current_count
Use java code below to generate fake optimization data(to be used in start page):
1 | public static void main(String[] args) throws IOException { |
Usage
Each field means the threshold of each component except wave. In the case of wave, the threshold needs to be pre-calculated using $H^2T$. User usage is in kwh per day or unit time, the unit time refers to the refresh frequency, which will be used to calculate energy produced in the frame of time.
The way to calculate energy produced per unit time: a KWH = b KW*s/3600, a is the result, b is the current power, a is the calculated energy. s is the refresh frequency. Refresh frequency in this form is in ms(milliseconds).
In the manual setting, you are able to choose to provide user usage data in the future, usually this means to have a column of data recording user consumption per unit time, it will be used as a source of consumming power. If that file doesn't have user usage, you could set it at here. In the auto generate mode, you have to provide one to let us find the optimal solution(guess a possible one would be fine).
Calculation Equation
Wave: $P_{total}=0.6*11*H^2T=6.6H^2T$ H - height of wave T - period
Ocean Current: $P=\frac{\rho v^3 S C_p}{2}=\frac{1050 v^3 \pi1.3^2* 0.45}{2}=1254v^3$ P is the energy captured by the unit, ρ is the fluid density, V is the fluid velocity, and S is the swept area of the impeller$C_p$ is the energy utilization coefficient
Light: $P=\frac{798*H_A}{365*24}=0.09*H_A, H_A$ Total solar radiation per unit area
Wind: $P=\frac{\rho v^3 S C_p}{2}=\frac{1.293 v^3 9852* 0.45}{2}=2866v^3$ P is the energy captured by the unit, ρ is the fluid density, V is the fluid velocity, and S is the swept area of the impeller$C_p$ is the energy utilization coefficient
Sample running
Optimization is done automatically and is not shown in this demo.
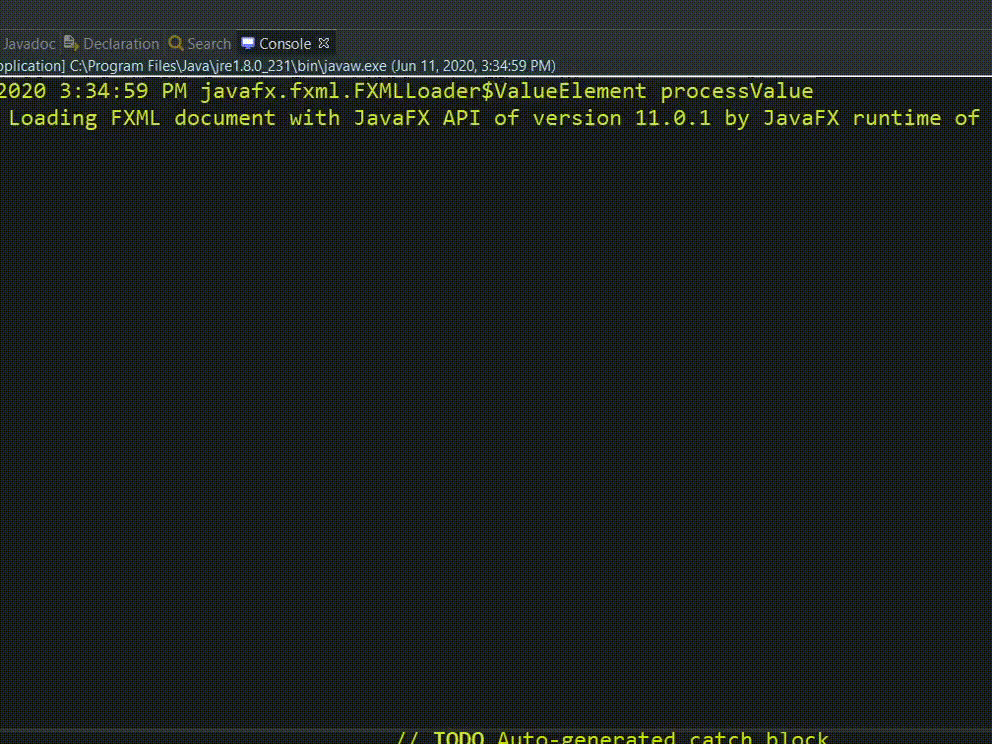